Following are the implementations of simple Breadth First Traversal from a given source.
The implementation uses adjacency list representation of graphs. STL‘s list container is used to store lists of adjacent nodes and queue of nodes needed for BFS traversal.
- C++
- Java
- Python3
Output:
Following is Breadth First Traversal (starting from vertex 2) 2 0 3 1
Illustration :
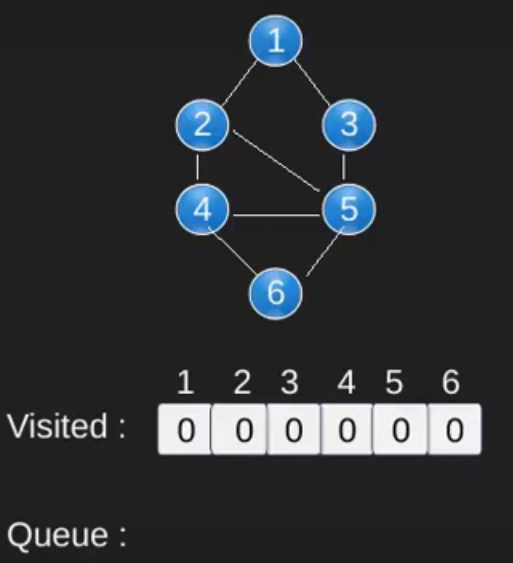
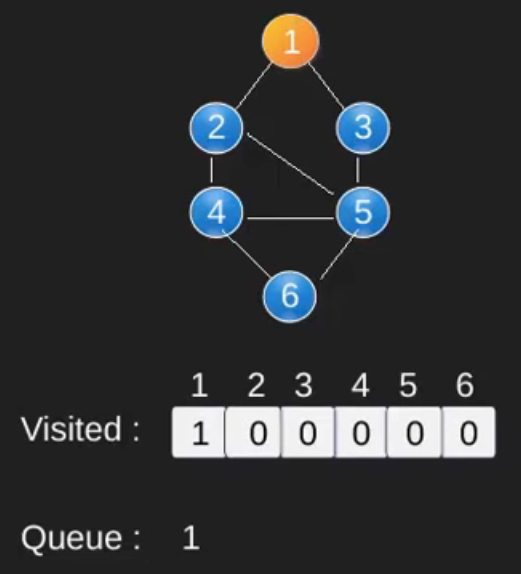
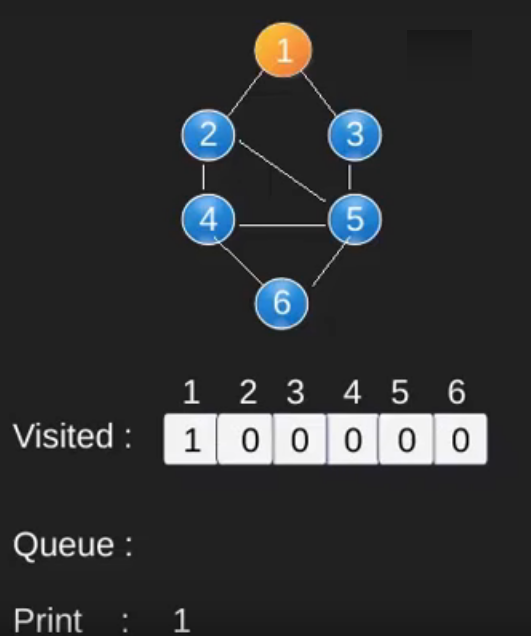
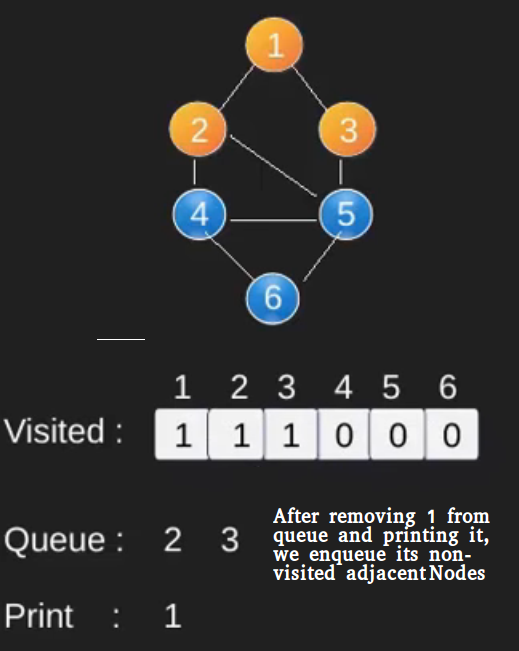
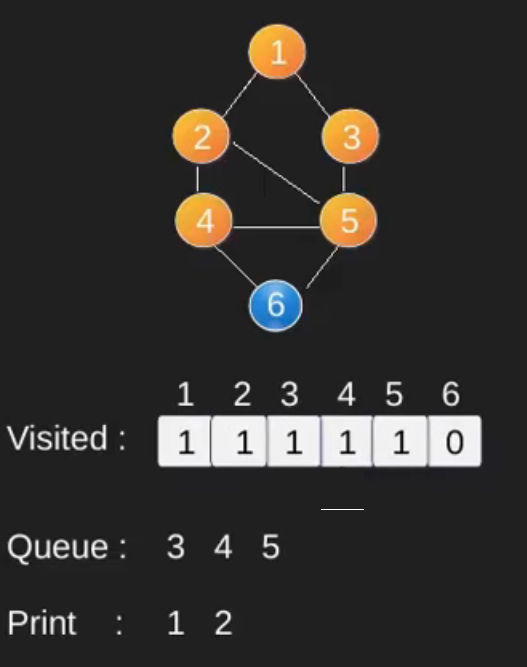
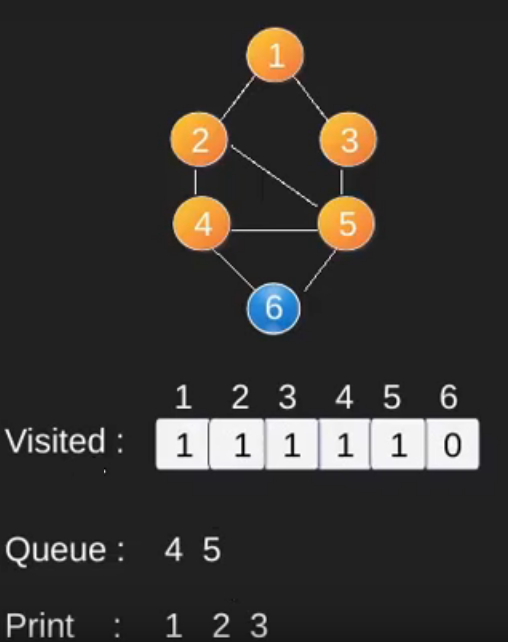
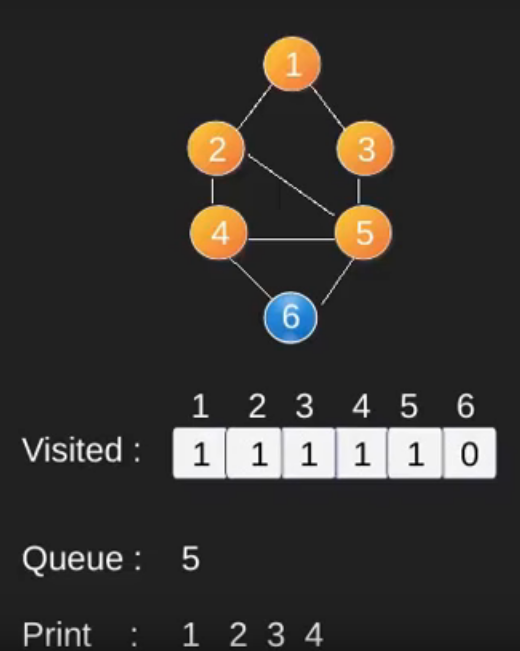
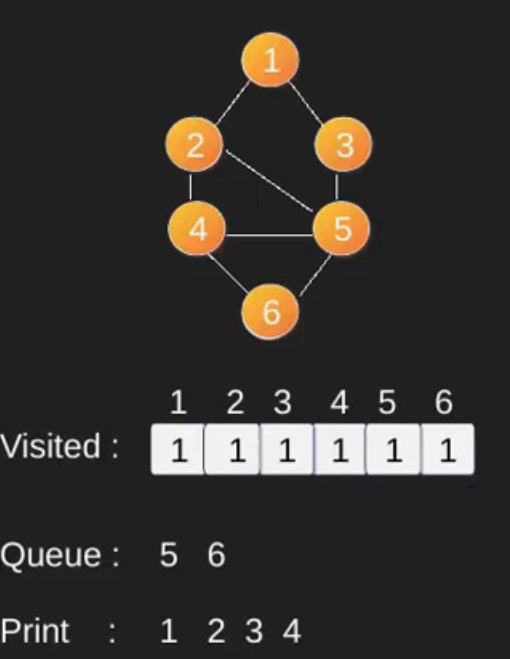
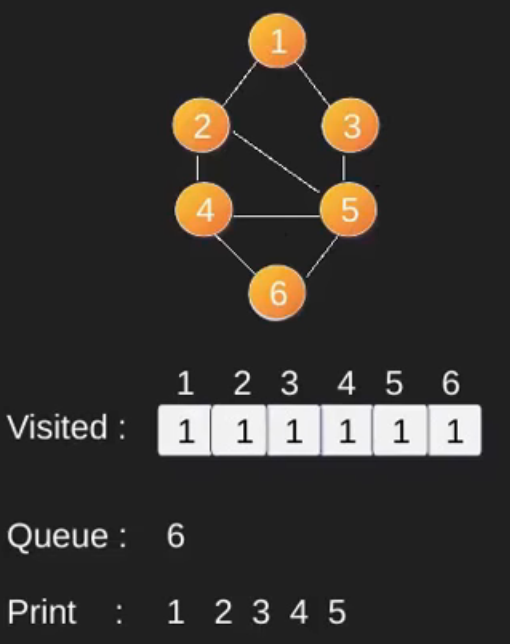
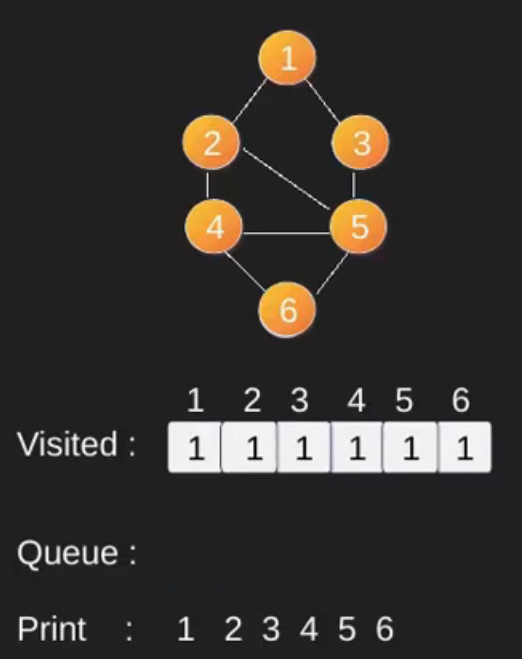
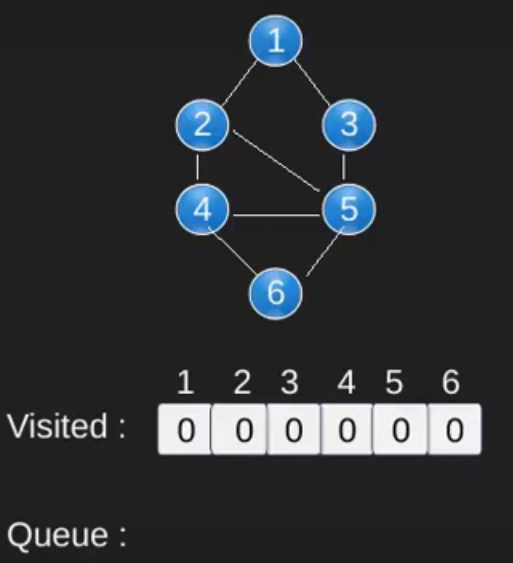
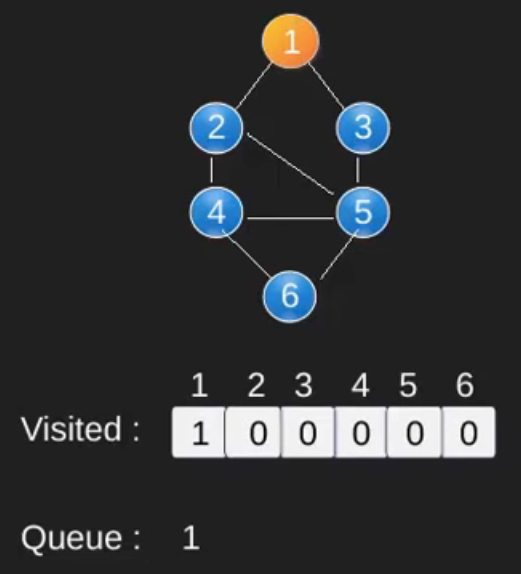
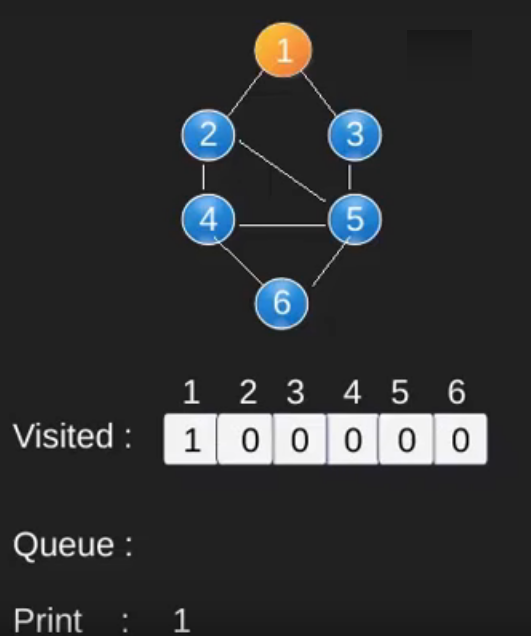
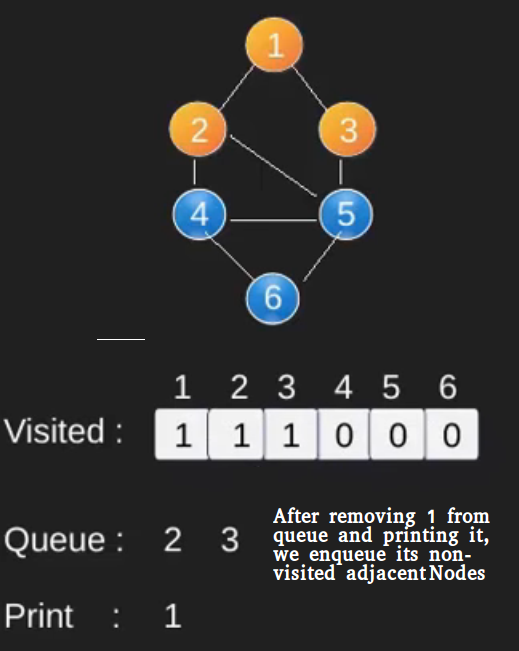
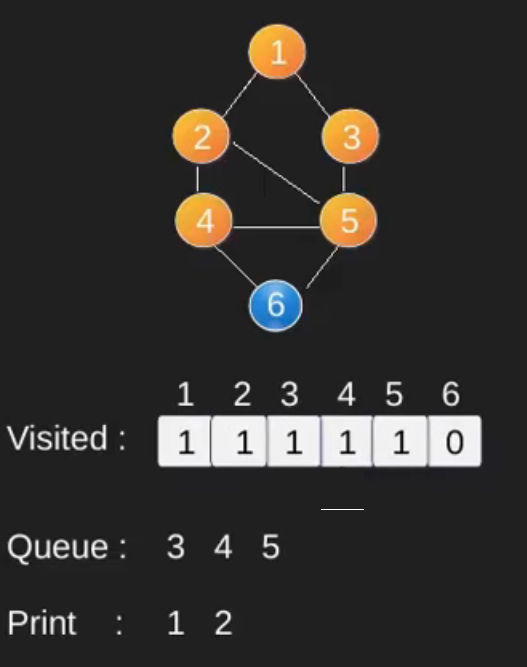
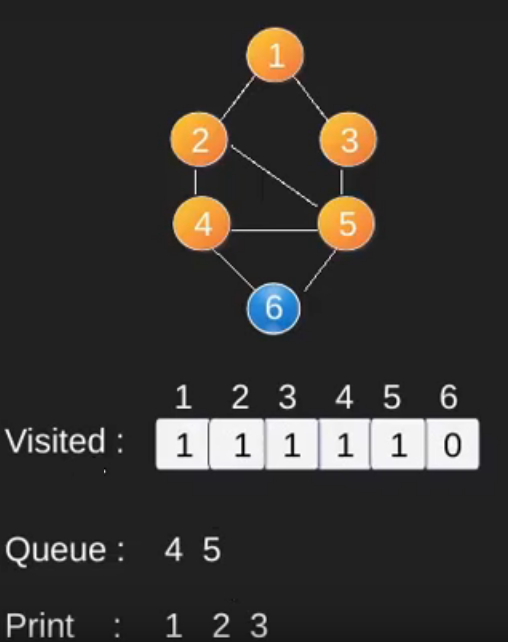
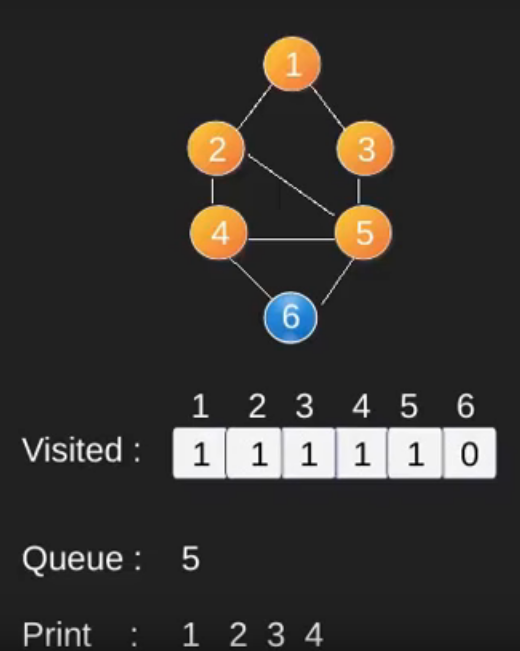
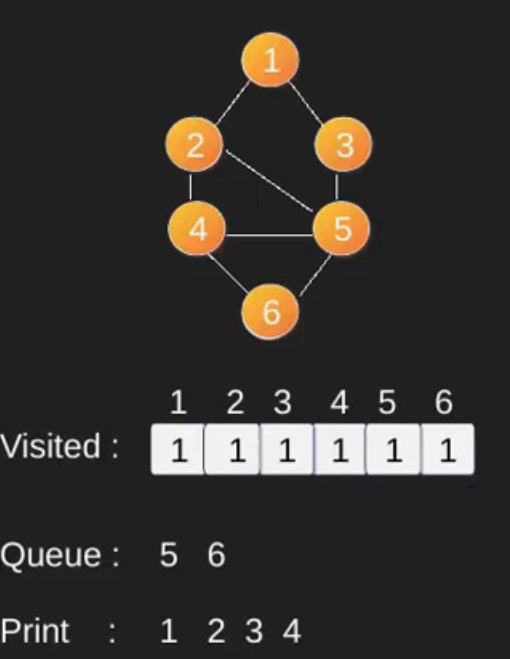
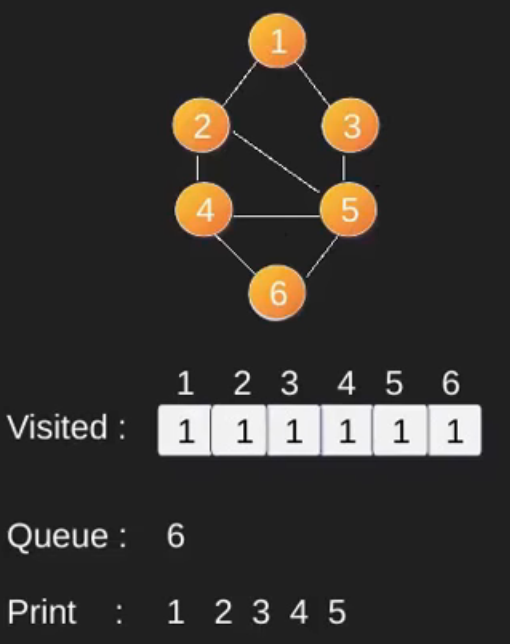
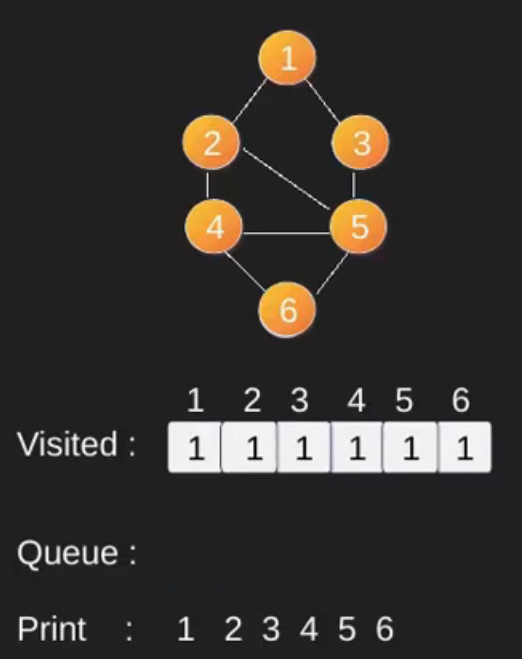
Note that the above code traverses only the vertices reachable from a given source vertex. All the vertices may not be reachable from a given vertex (example Disconnected graph). To print all the vertices, we can modify the BFS function to do traversal starting from all nodes one by one (Like the DFS modified version) .
Time Complexity: O(V+E) where V is number of vertices in the graph and E is number of edges in the graph.
Comments
Post a Comment